SwiftUI's native bottom sheet component is excellent and usually requires minimal effort. However, there are times when calculating the exact height can be tedious. In this post, we explore a way to make the bottom sheet dynamically adjust its height based on its content.
Problem Overview
Starting with the code below, we have a simple button that triggers a bottom sheet to appear on the screen using the .sheet
modifier in SwiftUI. We've set the sheet's height to the medium value, which roughly places it in the middle of the screen. However, this is only an approximation and not the exact middle.
swiftstruct ContentView: View { @State private var showSheet: Bool = false var body: some View { Button { showSheet.toggle() } label: { Image(systemName: "plus.circle") .font(.system(size: 40)) .foregroundStyle(.tint) } .sheet(isPresented: $showSheet) { sheetContentView .presentationBackground(.ultraThinMaterial) .presentationDragIndicator(.visible) .presentationDetents([.medium]) } } private var sheetContentView: some View { ZStack { Color.black.opacity(0.2).ignoresSafeArea() VStack { ForEach(0..<10) { value in Text("Hi there!") } } .padding(20) } } }
This code will output the following result:
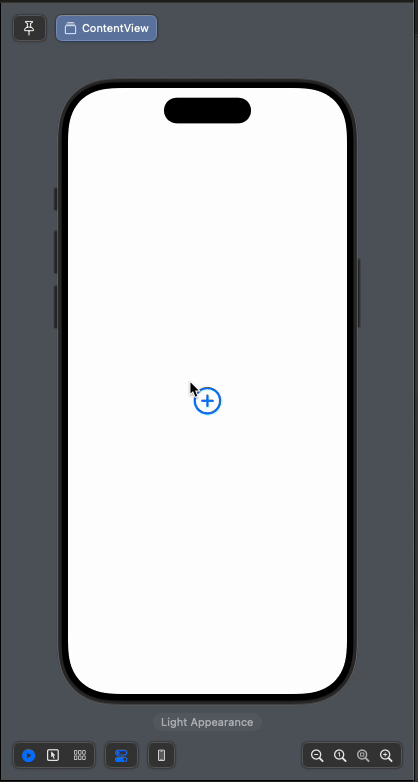
But if we change the number of Text
views like this:
swiftprivate var sheetContentView: some View { ZStack { Color.black.opacity(0.2).ignoresSafeArea() VStack { ForEach(0..<40) { value in Text("Hi there!") } } .padding(20) } }
Our content will be cropped and overflow:
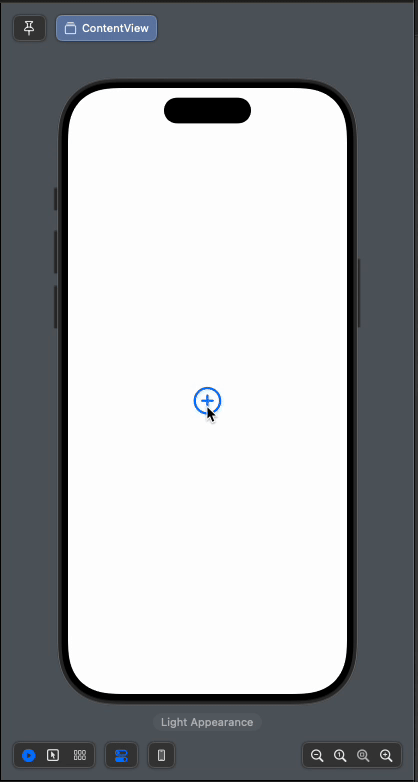
Of course, this is an exaggeration, and there are likely multiple ways to address this, with a ScrollView
being the most obvious solution. However, in this article, we’ll focus on the basic approach for scenarios where you need an image or certain views that can't have a hardcoded height value.
In this case, the medium detent height is considered a hardcoded value and is fixed. Any content that exceeds this value will cause it to overflow.
Proposed solution
The easiest approach that works in many cases is to use a combination of GeometryReader, preference keys, view modifiers, and view extensions.
1. Create a custom PreferenceKey
swiftstruct AdaptableHeightPreferenceKey: PreferenceKey { static var defaultValue: CGFloat? static func reduce(value: inout CGFloat?, nextValue: () -> CGFloat?) { guard let nextValue = nextValue() else { return } value = nextValue } }
This code defines a custom PreferenceKey called AdaptableHeightPreferenceKey
to store and update a CGFloat
value. It allows views to communicate their height to parent views by passing the height through the PreferenceKey
mechanism, enabling dynamic adjustments based on the content.
2. Create a custom View Modifier
swiftstruct AdaptableHeightModifier: ViewModifier { @Binding var currentHeight: CGFloat private var sizeView: some View { GeometryReader { geometry in Color.clear .preference(key: AdaptableHeightPreferenceKey.self, value: geometry.size.height) } } func body(content: Content) -> some View { content .background(sizeView) .onPreferenceChange(AdaptableHeightPreferenceKey.self) { height in if let height { currentHeight = height } } } }
Here, we define a ViewModifier called AdaptableHeightModifier
that dynamically adjusts a view's height based on its content.
It uses a GeometryReader
to measure the height of the view and passes the value using a PreferenceKey
. The modifier listens for changes to this value and updates the currentHeight binding with the new height, enabling adaptive resizing of the view.
By placing the GeometryReader
in the background with Color.clear
ensures that it doesn't interfere with the layout or structure of the view.
3. Create a View extension
swiftextension View { func readAndBindHeight(to height: Binding<CGFloat>) -> some View { self.modifier(AdaptableHeightModifier(currentHeight: height)) } }
Above, we created an extension for View
that adds a method called readAndBindHeight(to:)
.
When you call this method on a view, it applies the AdaptableHeightModifier
, which measures the height of the view and binds that height to a Binding<CGFloat>
. This allows the view's height to be dynamically tracked and updated outside of the view itself.
Now we just need to add another @State
variable to our view just like this:
swift@State private var detentHeight: CGFloat = 0
And then use this new modifier on our bottom sheet with the height detent this time:
swift.sheet(isPresented: $showSheet) { sheetContentView .presentationBackground(.ultraThinMaterial) .presentationDragIndicator(.visible) .presentationDetents([.height(detentHeight)]) .readAndBindHeight(to: $detentHeight) }
And that's it! Now, the bottom sheet height will dynamically adjust based on the content inside it.
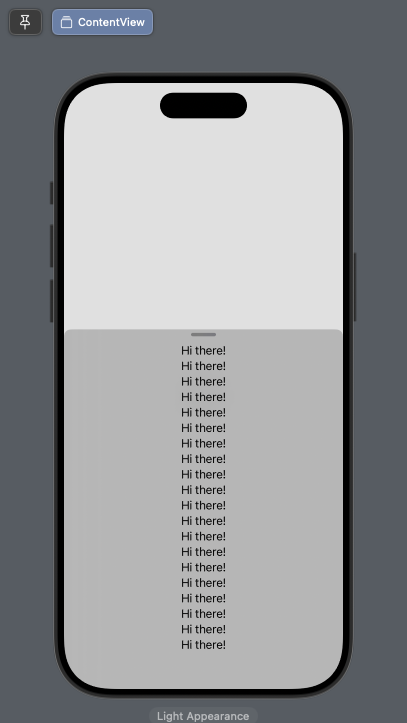
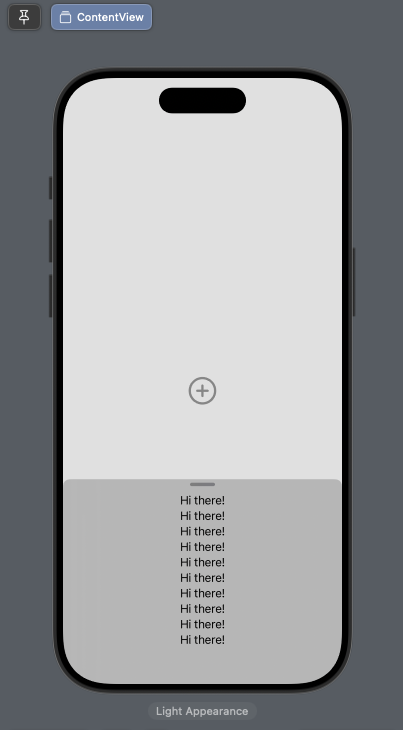